002 - Raspberry Pi Pico: LCD 4-bit Mode
Introduction
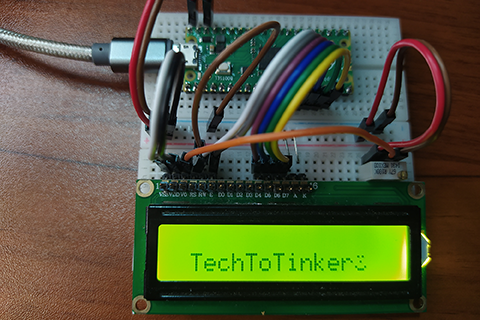
In this article, we will learn on how to use the 16×2 LCD module in 4-bit Mode with MicroPython language. For this tutorial, we will used a Raspberry Pi Pico with MicroPython firmware.
Bill Of Materials
- Raspberry Pi Pico development board.
- 16×2 LCD module the ordinary one without I2C interface.
- Breadboard to hold and connect the circuit.
- Jumper wires to connect the circuit.
Circuit Diagram
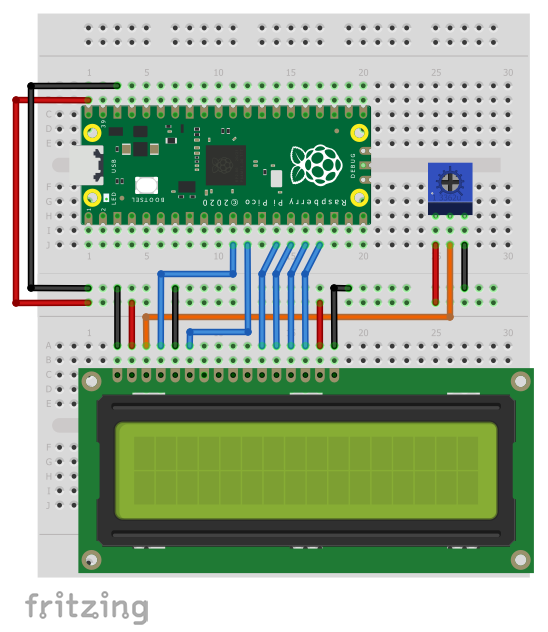
Hardware Instruction
- Connect the LCD VSS pin to the ground.
- Connect the LCD VDD pin to the 5V pin.
- Connect the LCD VO pin at the center wiper of the potentiometer.
- Connect the LCD RS pin to GPIO 8 of the Pico.
- Connect the LCD RW pin to the ground pin.
- Connect the LCD E pin to the GPIO 9 of the Pico.
- Connect the LCD D4 pin to the GPIO 10 of the Pico.
- Connect the LCD D5 pin to the GPIO 11 of the Pico.
- Connect the LCD D6 pin to the GPIO 12 of the Pico.
- Connect the LCD D7 pin to the GPIO 13 of the Pico.
Software Instruction
- Copy the lcd_api.py and the gpio_lcd.py to the Raspberry Pi Pico MicroPython root directory.
- Try the sample source code and adapt it according to your needs.
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example source code for exploring the basic functions:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin
5from gpio_lcd import GpioLcd
6
7# Create the LCD object
8lcd = GpioLcd(rs_pin=Pin(8),
9 enable_pin=Pin(9),
10 d4_pin=Pin(10),
11 d5_pin=Pin(11),
12 d6_pin=Pin(12),
13 d7_pin=Pin(13),
14 num_lines=2, num_columns=16)
15
16
17
18# #The following line of codes should be tested using the REPL
19#
20# #1. To print a string to the lcd, you can use
21lcd.putstr('Hello world!')
22# #2. Now, to clear the display.
23# lcd.clear()
24# #3. and to exactly position the cursor location
25# lcd.move_to(1,1)
26# lcd.putstr('TechToTinker')
27# # If you do not set the cursor position,
28# # the character will be displayed in the
29# # default cursor position starting from
30# # 0, x and 0, y location which is the top left-hand side.
31# # There are other useful functions we can use in using the lcd.
32# #4. Show the cursor
33# lcd.show_cursor()
34# #5. Hide the cursor
35# lcd.hide_cursor()
36# #6. Turn ON blinking cursor
37# lcd.blink_cursor_on()
38# #7. Turn OFF blinking cursor
39# lcd.blink_cursor_off()
40# #8. Disable display
41# lcd.display_off()
42# this will only hide the characters
43# #9. Enable display
44# lcd.display_on()
45# #10. Turn backlight OFF
46# lcd.backlight_off()
47# #11. Turn backlight ON
48# lcd.backlight_on()
49# # 12. Print a single character
50# lcd.putchar('x')
51# but this will only print 1 character
52# #13. Display a custom characters
53# happy_face = bytearray([0x00,0x0A,0x00,0x04,0x00,0x11,0x0E,0x00])
54# lcd.custom_char(0, happy_face)
55# lcd.putchar(chr(0))
py
2. lcd_api.py :
1"""Provides an API for talking to HD44780 compatible character LCDs."""
2
3import time
4
5class LcdApi:
6 """Implements the API for talking with HD44780 compatible character LCDs.
7 This class only knows what commands to send to the LCD, and not how to get
8 them to the LCD.
9 It is expected that a derived class will implement the hal_xxx functions.
10 """
11
12 # The following constant names were lifted from the avrlib lcd.h
13 # header file, however, I changed the definitions from bit numbers
14 # to bit masks.
15 #
16 # HD44780 LCD controller command set
17
18 LCD_CLR = 0x01 # DB0: clear display
19 LCD_HOME = 0x02 # DB1: return to home position
20
21 LCD_ENTRY_MODE = 0x04 # DB2: set entry mode
22 LCD_ENTRY_INC = 0x02 # --DB1: increment
23 LCD_ENTRY_SHIFT = 0x01 # --DB0: shift
24
25 LCD_ON_CTRL = 0x08 # DB3: turn lcd/cursor on
26 LCD_ON_DISPLAY = 0x04 # --DB2: turn display on
27 LCD_ON_CURSOR = 0x02 # --DB1: turn cursor on
28 LCD_ON_BLINK = 0x01 # --DB0: blinking cursor
29
30 LCD_MOVE = 0x10 # DB4: move cursor/display
31 LCD_MOVE_DISP = 0x08 # --DB3: move display (0-> move cursor)
32 LCD_MOVE_RIGHT = 0x04 # --DB2: move right (0-> left)
33
34 LCD_FUNCTION = 0x20 # DB5: function set
35 LCD_FUNCTION_8BIT = 0x10 # --DB4: set 8BIT mode (0->4BIT mode)
36 LCD_FUNCTION_2LINES = 0x08 # --DB3: two lines (0->one line)
37 LCD_FUNCTION_10DOTS = 0x04 # --DB2: 5x10 font (0->5x7 font)
38 LCD_FUNCTION_RESET = 0x30 # See "Initializing by Instruction" section
39
40 LCD_CGRAM = 0x40 # DB6: set CG RAM address
41 LCD_DDRAM = 0x80 # DB7: set DD RAM address
42
43 LCD_RS_CMD = 0
44 LCD_RS_DATA = 1
45
46 LCD_RW_WRITE = 0
47 LCD_RW_READ = 1
48
49 def __init__(self, num_lines, num_columns):
50 self.num_lines = num_lines
51 if self.num_lines > 4:
52 self.num_lines = 4
53 self.num_columns = num_columns
54 if self.num_columns > 40:
55 self.num_columns = 40
56 self.cursor_x = 0
57 self.cursor_y = 0
58 self.implied_newline = False
59 self.backlight = True
60 self.display_off()
61 self.backlight_on()
62 self.clear()
63 self.hal_write_command(self.LCD_ENTRY_MODE | self.LCD_ENTRY_INC)
64 self.hide_cursor()
65 self.display_on()
66
67 def clear(self):
68 """Clears the LCD display and moves the cursor to the top left
69 corner.
70 """
71 self.hal_write_command(self.LCD_CLR)
72 self.hal_write_command(self.LCD_HOME)
73 self.cursor_x = 0
74 self.cursor_y = 0
75
76 def show_cursor(self):
77 """Causes the cursor to be made visible."""
78 self.hal_write_command(self.LCD_ON_CTRL | self.LCD_ON_DISPLAY |
79 self.LCD_ON_CURSOR)
80
81 def hide_cursor(self):
82 """Causes the cursor to be hidden."""
83 self.hal_write_command(self.LCD_ON_CTRL | self.LCD_ON_DISPLAY)
84
85 def blink_cursor_on(self):
86 """Turns on the cursor, and makes it blink."""
87 self.hal_write_command(self.LCD_ON_CTRL | self.LCD_ON_DISPLAY |
88 self.LCD_ON_CURSOR | self.LCD_ON_BLINK)
89
90 def blink_cursor_off(self):
91 """Turns on the cursor, and makes it no blink (i.e. be solid)."""
92 self.hal_write_command(self.LCD_ON_CTRL | self.LCD_ON_DISPLAY |
93 self.LCD_ON_CURSOR)
94
95 def display_on(self):
96 """Turns on (i.e. unblanks) the LCD."""
97 self.hal_write_command(self.LCD_ON_CTRL | self.LCD_ON_DISPLAY)
98
99 def display_off(self):
100 """Turns off (i.e. blanks) the LCD."""
101 self.hal_write_command(self.LCD_ON_CTRL)
102
103 def backlight_on(self):
104 """Turns the backlight on.
105 This isn't really an LCD command, but some modules have backlight
106 controls, so this allows the hal to pass through the command.
107 """
108 self.backlight = True
109 self.hal_backlight_on()
110
111 def backlight_off(self):
112 """Turns the backlight off.
113 This isn't really an LCD command, but some modules have backlight
114 controls, so this allows the hal to pass through the command.
115 """
116 self.backlight = False
117 self.hal_backlight_off()
118
119 def move_to(self, cursor_x, cursor_y):
120 """Moves the cursor position to the indicated position. The cursor
121 position is zero based (i.e. cursor_x == 0 indicates first column).
122 """
123 self.cursor_x = cursor_x
124 self.cursor_y = cursor_y
125 addr = cursor_x & 0x3f
126 if cursor_y & 1:
127 addr += 0x40 # Lines 1 & 3 add 0x40
128 if cursor_y & 2: # Lines 2 & 3 add number of columns
129 addr += self.num_columns
130 self.hal_write_command(self.LCD_DDRAM | addr)
131
132 def putchar(self, char):
133 """Writes the indicated character to the LCD at the current cursor
134 position, and advances the cursor by one position.
135 """
136 if char == 'n':
137 if self.implied_newline:
138 # self.implied_newline means we advanced due to a wraparound,
139 # so if we get a newline right after that we ignore it.
140 pass
141 else:
142 self.cursor_x = self.num_columns
143 else:
144 self.hal_write_data(ord(char))
145 self.cursor_x += 1
146 if self.cursor_x >= self.num_columns:
147 self.cursor_x = 0
148 self.cursor_y += 1
149 self.implied_newline = (char != 'n')
150 if self.cursor_y >= self.num_lines:
151 self.cursor_y = 0
152 self.move_to(self.cursor_x, self.cursor_y)
153
154 def putstr(self, string):
155 """Write the indicated string to the LCD at the current cursor
156 position and advances the cursor position appropriately.
157 """
158 for char in string:
159 self.putchar(char)
160
161 def custom_char(self, location, charmap):
162 """Write a character to one of the 8 CGRAM locations, available
163 as chr(0) through chr(7).
164 """
165 location &= 0x7
166 self.hal_write_command(self.LCD_CGRAM | (location << 3))
167 self.hal_sleep_us(40)
168 for i in range(8):
169 self.hal_write_data(charmap[i])
170 self.hal_sleep_us(40)
171 self.move_to(self.cursor_x, self.cursor_y)
172
173 def hal_backlight_on(self):
174 """Allows the hal layer to turn the backlight on.
175 If desired, a derived HAL class will implement this function.
176 """
177 pass
178
179 def hal_backlight_off(self):
180 """Allows the hal layer to turn the backlight off.
181 If desired, a derived HAL class will implement this function.
182 """
183 pass
184
185 def hal_write_command(self, cmd):
186 """Write a command to the LCD.
187 It is expected that a derived HAL class will implement this
188 function.
189 """
190 raise NotImplementedError
191
192 def hal_write_data(self, data):
193 """Write data to the LCD.
194 It is expected that a derived HAL class will implement this
195 function.
196 """
197 raise NotImplementedError
198
199 def hal_sleep_us(self, usecs):
200 """Sleep for some time (given in microseconds)."""
201 time.sleep_us(usecs)
py
3. gpio_lcd.py :
1"""Implements a HD44780 character LCD connected via ESP32 GPIO pins."""
2
3from lcd_api import LcdApi
4from machine import Pin
5from utime import sleep_ms, sleep_us
6
7
8class GpioLcd(LcdApi):
9 """Implements a HD44780 character LCD connected via ESP32 GPIO pins."""
10
11 def __init__(self, rs_pin, enable_pin, d0_pin=None, d1_pin=None,
12 d2_pin=None, d3_pin=None, d4_pin=None, d5_pin=None,
13 d6_pin=None, d7_pin=None, rw_pin=None, backlight_pin=None,
14 num_lines=2, num_columns=16):
15 """Constructs the GpioLcd object. All of the arguments must be machine.Pin
16 objects which describe which pin the given line from the LCD is
17 connected to.
18 When used in 4-bit mode, only D4, D5, D6, and D7 are physically
19 connected to the LCD panel. This function allows you call it like
20 GpioLcd(rs, enable, D4, D5, D6, D7) and it will interpret that as
21 if you had actually called:
22 GpioLcd(rs, enable, d4=D4, d5=D5, d6=D6, d7=D7)
23 The enable 8-bit mode, you need pass d0 through d7.
24 The rw pin isn't used by this library, but if you specify it, then
25 it will be set low.
26 """
27 self.rs_pin = rs_pin
28 self.enable_pin = enable_pin
29 self.rw_pin = rw_pin
30 self.backlight_pin = backlight_pin
31 self._4bit = True
32 if d4_pin and d5_pin and d6_pin and d7_pin:
33 self.d0_pin = d0_pin
34 self.d1_pin = d1_pin
35 self.d2_pin = d2_pin
36 self.d3_pin = d3_pin
37 self.d4_pin = d4_pin
38 self.d5_pin = d5_pin
39 self.d6_pin = d6_pin
40 self.d7_pin = d7_pin
41 if self.d0_pin and self.d1_pin and self.d2_pin and self.d3_pin:
42 self._4bit = False
43 else:
44 # This is really 4-bit mode, and the 4 data pins were just
45 # passed as the first 4 arguments, so we switch things around.
46 self.d0_pin = None
47 self.d1_pin = None
48 self.d2_pin = None
49 self.d3_pin = None
50 self.d4_pin = d0_pin
51 self.d5_pin = d1_pin
52 self.d6_pin = d2_pin
53 self.d7_pin = d3_pin
54 self.rs_pin.init(Pin.OUT)
55 self.rs_pin.value(0)
56 if self.rw_pin:
57 self.rw_pin.init(Pin.OUT)
58 self.rw_pin.value(0)
59 self.enable_pin.init(Pin.OUT)
60 self.enable_pin.value(0)
61 self.d4_pin.init(Pin.OUT)
62 self.d5_pin.init(Pin.OUT)
63 self.d6_pin.init(Pin.OUT)
64 self.d7_pin.init(Pin.OUT)
65 self.d4_pin.value(0)
66 self.d5_pin.value(0)
67 self.d6_pin.value(0)
68 self.d7_pin.value(0)
69 if not self._4bit:
70 self.d0_pin.init(Pin.OUT)
71 self.d1_pin.init(Pin.OUT)
72 self.d2_pin.init(Pin.OUT)
73 self.d3_pin.init(Pin.OUT)
74 self.d0_pin.value(0)
75 self.d1_pin.value(0)
76 self.d2_pin.value(0)
77 self.d3_pin.value(0)
78 if self.backlight_pin is not None:
79 self.backlight_pin.init(Pin.OUT)
80 self.backlight_pin.value(0)
81
82 # See about splitting this into begin
83
84 sleep_ms(20) # Allow LCD time to powerup
85 # Send reset 3 times
86 self.hal_write_init_nibble(self.LCD_FUNCTION_RESET)
87 sleep_ms(5) # need to delay at least 4.1 msec
88 self.hal_write_init_nibble(self.LCD_FUNCTION_RESET)
89 sleep_ms(1)
90 self.hal_write_init_nibble(self.LCD_FUNCTION_RESET)
91 sleep_ms(1)
92 cmd = self.LCD_FUNCTION
93 if not self._4bit:
94 cmd |= self.LCD_FUNCTION_8BIT
95 self.hal_write_init_nibble(cmd)
96 sleep_ms(1)
97 LcdApi.__init__(self, num_lines, num_columns)
98 if num_lines > 1:
99 cmd |= self.LCD_FUNCTION_2LINES
100 self.hal_write_command(cmd)
101
102 def hal_pulse_enable(self):
103 """Pulse the enable line high, and then low again."""
104 self.enable_pin.value(0)
105 sleep_us(1)
106 self.enable_pin.value(1)
107 sleep_us(1) # Enable pulse needs to be > 450 nsec
108 self.enable_pin.value(0)
109 sleep_us(100) # Commands need > 37us to settle
110
111 def hal_write_init_nibble(self, nibble):
112 """Writes an initialization nibble to the LCD.
113 This particular function is only used during initialization.
114 """
115 self.hal_write_4bits(nibble >> 4)
116
117 def hal_backlight_on(self):
118 """Allows the hal layer to turn the backlight on."""
119 if self.backlight_pin:
120 self.backlight_pin.value(1)
121
122 def hal_backlight_off(self):
123 """Allows the hal layer to turn the backlight off."""
124 if self.backlight_pin:
125 self.backlight_pin.value(0)
126
127 def hal_write_command(self, cmd):
128 """Writes a command to the LCD.
129 Data is latched on the falling edge of E.
130 """
131 self.rs_pin.value(0)
132 self.hal_write_8bits(cmd)
133 if cmd <= 3:
134 # The home and clear commands require a worst
135 # case delay of 4.1 msec
136 sleep_ms(5)
137
138 def hal_write_data(self, data):
139 """Write data to the LCD."""
140 self.rs_pin.value(1)
141 self.hal_write_8bits(data)
142
143 def hal_write_8bits(self, value):
144 """Writes 8 bits of data to the LCD."""
145 if self.rw_pin:
146 self.rw_pin.value(0)
147 if self._4bit:
148 self.hal_write_4bits(value >> 4)
149 self.hal_write_4bits(value)
150 else:
151 self.d3_pin.value(value & 0x08)
152 self.d2_pin.value(value & 0x04)
153 self.d1_pin.value(value & 0x02)
154 self.d0_pin.value(value & 0x01)
155 self.hal_write_4bits(value >> 4)
156
157 def hal_write_4bits(self, nibble):
158 """Writes 4 bits of data to the LCD."""
159 self.d7_pin.value(nibble & 0x08)
160 self.d6_pin.value(nibble & 0x04)
161 self.d5_pin.value(nibble & 0x02)
162 self.d4_pin.value(nibble & 0x01)
163 self.hal_pulse_enable()
py
References And Credits
- Dave Hylands LCD library: https://github.com/dhylands/python_lcd
Posts in this series
- 003 - Raspberry Pi Pico: Charlieplexing
- 001 - Raspberry Pi Pico: GPIO | MicroPython
- 000 - Raspberry Pi Pico: How to get started | MicroPython
No comments yet!