005 - ESP32 MicroPython: Pulse Width Modulation
Introduction
In this tutorial, we will learn to use the PWM of ESP32 in MicroPython. PWM stands for Pulse Width Modulation.
Circuit Diagram
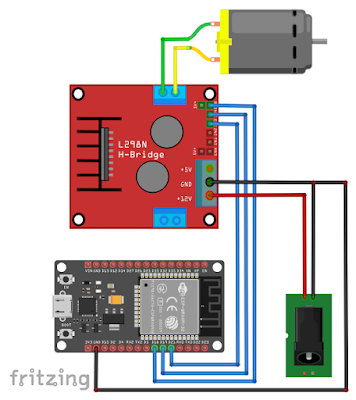
Video Demonstration
Call To Action
If you find this tutorial helpful, please consider Subscribing to my Youtube channel by clicking this link to SUBSCRIBE to TechToTinker Youtube channel.
Thank you.
Source Code
1# DC motor speed control using PWM in MicroPython
2# Author: George Bantique, TechToTinker
3# Date: September 13, 2020
4
5# Load the machine module which also
6# includes the pwm class
7import machine
8
9# Create the drives object for the direction
10# of rotation of the DC motor
11dr1 = machine.Pin(21, machine.Pin.OUT)
12dr2 = machine.Pin(19, machine.Pin.OUT)
13
14# Create the en1 object as normal GPIO
15en1 = machine.Pin(18, machine.Pin.OUT)
16
17# Create a pwm object and attach it to
18# pwm drivers
19pwm = machine.PWM(en1)
20
21# Rotates the DC motor clockwise
22def cw():
23 dr1.value(1)
24 dr2.value(0)
25
26# Rotates the DC motor counter-clockwise
27def ccw():
28 dr1.value(0)
29 dr2.value(1)
30
31# Starts the pwm with a definite value
32# and start the motor rotating according
33# to the input argument
34def start(rotation):
35 pwm.init(freq=1, duty=512)
36 if (rotation=='cw'):
37 cw()
38 elif (rotation=='ccw'):
39 ccw()
40
41# Stops the pwm using pwm.deinit() function
42# which dettach the pwm driver to the en1 object
43# We also provide the drives pin a logic low value
44def stop():
45 pwm.deinit()
46 dr1.value(0)
47 dr2.value(0)
48
py
Posts in this series
- 026 - ESP32 MicroPython: MFRC522 RFID Module
- 025 - ESP32 MicroPython: ESP32 Bluetooth Low Energy
- 024 - ESP32 MicroPython: How to Use SD Card in MicroPython
- 023 - ESP32 MicroPython: Binary Clock
- 022 - ESP32 MicroPython: MQTT Part 2: Subscribe
- 021 - ESP32 MicroPython: MQTT Part 1: Publish
- 020 - ESP32 MicroPython: RESTful APIs | Demo READ and WRITE
- 019 - ESP32 MicroPython: OpenWeather | RESTful APIs
- 018 - ESP32 MicroPython: Thingspeak | RESTful APIs
- 017 - ESP32 MicroPython: DHT Values Auto Updates using AJAX
- 016 - ESP32 MicroPython: Web Server | ESP32 Access Point
- 015 - ESP32 MicroPython: Web Server | ESP32 Station Mode in MicroPython
- 014 - ESP32 MicroPython: SIM800L GSM Module in MicroPython
- 013 - ESP32 MicroPython: UART Serial in MicroPython
- 012 - ESP32 MicroPython: HC-SR04 Ultrasonic Sensor in MicroPython
- 011 - ESP32 MicroPython: DHT11, DHT22 in MicroPython
- 010 - ESP32 MicroPython: 0.96 OLED in MicroPython
- 009 - ESP32 MicroPython: Non-blocking Delays and Multithreading | Multitasking
- 008 - ESP32 MicroPython: Hardware Timer Interrupts
- 007 - ESP32 MicroPython: How to make some sound with MicroPython
- 006 - ESP32 MicroPython: How to control servo motor with MicroPython
- 004 - ESP32 MicroPython: External Interrupts
- 003 - ESP32 MicroPython: General Purpose Input Output | GPIO Pins
- 001 - ESP32 MicroPython: What is MicroPython
- 000 - ESP32 MicroPython: How to Get Started with MicroPython
No comments yet!