016 - ESP32 MicroPython: Web Server | ESP32 Access Point
Introduction
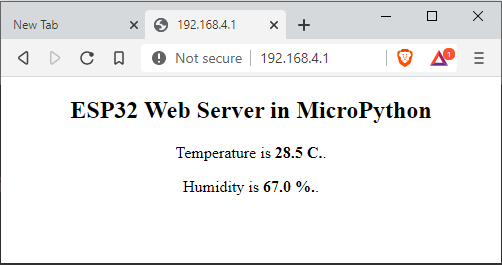
In this article you will learn to configure ESP32 wifi as Access Point. You will also learn to create a web server hosted on it.
Bill Of Materials
- ESP32 development board.
- DHT22 (or DHT11 with slight, very small modification in the code).
- Connecting wires.
Hardware Instruction
- Connect the DHT22 VCC pin to 3.3V pin of ESP32.
- Connect the DHT22 Data pin to D23 pin of ESP32.
- Connect the DHT22 GND pin to GND pin of ESP32.

Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example # 1, Web Server on ESP32 as Wifi Access Point. The web server is use for controlling the GPIO state of the pins.
1# ************************
2#
3import machine
4import time
5led = machine.Pin(2,machine.Pin.OUT)
6led.off()
7
8
9# ************************
10# Configure the ESP32 wifi
11# as Access Point mode.
12import network
13ssid = 'ESP32-AP-WebServer'
14password = '123456789'
15
16ap = network.WLAN(network.AP_IF)
17ap.active(True)
18ap.config(essid=ssid, password=password)
19while not ap.active():
20 pass
21print('network config:', ap.ifconfig())
22
23# ************************
24# Configure the socket connection
25# over TCP/IP
26import socket
27
28# AF_INET - use Internet Protocol v4 addresses
29# SOCK_STREAM means that it is a TCP socket.
30# SOCK_DGRAM means that it is a UDP socket.
31s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
32s.bind(('',80)) # specifies that the socket is reachable by any address the machine happens to have
33s.listen(5) # max of 5 socket connections
34
35# ************************
36# Function for creating the
37# web page to be displayed
38def web_page():
39 if led.value()==1:
40 led_state = 'ON'
41 print('led is ON')
42 elif led.value()==0:
43 led_state = 'OFF'
44 print('led is OFF')
45
46 html_page = """
47 <html>
48 <head>
49 <meta name="viewport" content="width=device-width, initial-scale=1">
50 </head>
51 <body>
52 <center><h2>ESP32 Web Server in MicroPython </h2></center>
53 <center>
54 <form>
55 <button type='submit' name="LED" value='1'> LED ON </button>
56 <button type='submit' name="LED" value='0'> LED OFF </button>
57 </form>
58 </center>
59 <center><p>LED is now <strong>""" + led_state + """</strong>.</p></center>
60 </body>
61 </html>"""
62 return html_page
63
64while True:
65 # Socket accept()
66 conn, addr = s.accept()
67 print("Got connection from %s" % str(addr))
68
69 # Socket receive()
70 request=conn.recv(1024)
71 print("")
72 print("")
73 print("Content %s" % str(request))
74
75 # Socket send()
76 request = str(request)
77 led_on = request.find('/?LED=1')
78 led_off = request.find('/?LED=0')
79 if led_on == 6:
80 print('LED ON')
81 print(str(led_on))
82 led.value(1)
83 elif led_off == 6:
84 print('LED OFF')
85 print(str(led_off))
86 led.value(0)
87 response = web_page()
88 conn.send('HTTP/1.1 200 OKn')
89 conn.send('Content-Type: text/htmln')
90 conn.send('Connection: close\n')
91 conn.sendall(response)
92
93 # Socket close()
94 conn.close()
py
2. Example # 2, Display DHT sensor readings through a web server:
1# ************************
2#
3import machine
4import time
5led = machine.Pin(2,machine.Pin.OUT)
6led.off()
7# ************************
8# Configure the ESP32 wifi
9# as Access Point mode.
10import network
11ssid = 'ESP32-AP-WebServer'
12password = '123456789'
13ap = network.WLAN(network.AP_IF)
14ap.active(True)
15ap.config(essid=ssid, password=password)
16while not ap.active():
17 pass
18print('network config:', ap.ifconfig())
19# ************************
20# Configure the socket connection
21# over TCP/IP
22import socket
23# AF_INET - use Internet Protocol v4 addresses
24# SOCK_STREAM means that it is a TCP socket.
25# SOCK_DGRAM means that it is a UDP socket.
26s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
27s.bind(('',80)) # specifies that the socket is reachable by any address the machine happens to have
28s.listen(5) # max of 5 socket connections
29# DHT sensor initializations
30import dht
31d = dht.DHT22(machine.Pin(23))
32# If you will use DHT11, change it to:
33# d = dht.DHT11(machine.Pin(23))
34# ************************
35# Function for creating the
36# web page to be displayed
37def web_page():
38 # Get the DHT readings
39 d.measure()
40 t = d.temperature()
41 h = d.humidity()
42
43 html_page = """
44 <html>
45 <head>
46 <meta name="viewport" content="width=device-width, initial-scale=1">
47 <meta http-equiv="refresh" content="1">
48 </head>
49 <body>
50 <center><h2>ESP32 Web Server in MicroPython </h2></center>
51 <center><p>Temperature is <strong>""" + str(t) + """ C.</strong>.</p></center>
52 <center><p>Humidity is <strong>""" + str(h) + """ %.</strong>.</p></center>
53 </body>
54 </html>"""
55 return html_page
56
57while True:
58 # Socket accept()
59 conn, addr = s.accept()
60 print("Got connection from %s" % str(addr))
61
62 # Socket receive()
63 request=conn.recv(1024)
64 print("")
65 print("Content %s" % str(request))
66 # Socket send()
67 request = str(request)
68
69 # Create a socket reply
70 response = web_page()
71 conn.send('HTTP/1.1 200 OKn')
72 conn.send('Content-Type: text/htmln')
73 conn.send('Connection: close\n')
74 conn.sendall(response)
75
76 # Socket close()
77 conn.close()
py
Posts in this series
- 026 - ESP32 MicroPython: MFRC522 RFID Module
- 025 - ESP32 MicroPython: ESP32 Bluetooth Low Energy
- 024 - ESP32 MicroPython: How to Use SD Card in MicroPython
- 023 - ESP32 MicroPython: Binary Clock
- 022 - ESP32 MicroPython: MQTT Part 2: Subscribe
- 021 - ESP32 MicroPython: MQTT Part 1: Publish
- 020 - ESP32 MicroPython: RESTful APIs | Demo READ and WRITE
- 019 - ESP32 MicroPython: OpenWeather | RESTful APIs
- 018 - ESP32 MicroPython: Thingspeak | RESTful APIs
- 017 - ESP32 MicroPython: DHT Values Auto Updates using AJAX
- 015 - ESP32 MicroPython: Web Server | ESP32 Station Mode in MicroPython
- 014 - ESP32 MicroPython: SIM800L GSM Module in MicroPython
- 013 - ESP32 MicroPython: UART Serial in MicroPython
- 012 - ESP32 MicroPython: HC-SR04 Ultrasonic Sensor in MicroPython
- 011 - ESP32 MicroPython: DHT11, DHT22 in MicroPython
- 010 - ESP32 MicroPython: 0.96 OLED in MicroPython
- 009 - ESP32 MicroPython: Non-blocking Delays and Multithreading | Multitasking
- 008 - ESP32 MicroPython: Hardware Timer Interrupts
- 007 - ESP32 MicroPython: How to make some sound with MicroPython
- 006 - ESP32 MicroPython: How to control servo motor with MicroPython
- 005 - ESP32 MicroPython: Pulse Width Modulation
- 004 - ESP32 MicroPython: External Interrupts
- 003 - ESP32 MicroPython: General Purpose Input Output | GPIO Pins
- 001 - ESP32 MicroPython: What is MicroPython
- 000 - ESP32 MicroPython: How to Get Started with MicroPython
No comments yet!