017 - ESP32 MicroPython: DHT Values Auto Updates using AJAX
Introduction
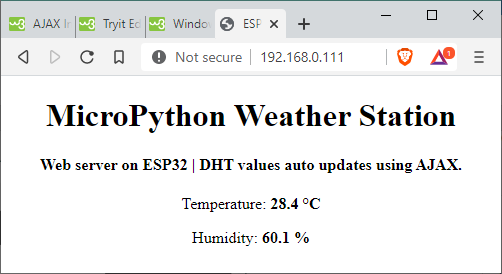
In the previous tutorials we learned to create web server both as station mode and access point mode using socket connection. And in last video, we use a DHT sensor for displaying measurement readings in simple web server similar to a weather station. While displaying sensor values, we discovered the necessity of constantly updating the displayed measurements. And we concluded that updating the whole web page using the html meta refresh is not efficient. I decided to learn AJAX in order to update only the DHT sensor values displayed without refreshing the whole web page.
Bill Of Materials
- ESP32 development board, or ESP8266.
- DHT22 temperature and humidity sensor, or DHT11.
Hardware Instruction
j
- Connect the DHT22 VCC pin to ESP32 3.3V pin.
- Connect the DHT22 Data pin to ESP32 D23 pin.
- Connect the DHT22 GND pin to ESP32 GND pin.
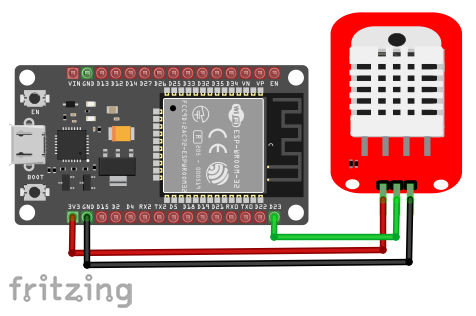
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1# ******************************************** #
2# DHT values auto updates using AJAX #
3# Author: George Bantique, TechToTinker #
4# tech.to.tinker@gmail.com #
5# Created: Nov. 11, 2020 #
6# #
7# NOTE: Please feel free to modify and adapt #
8# the source code according to your needs. #
9# #
10# ******************************************** #
11#
12import machine
13import dht
14led = machine.Pin(2,machine.Pin.OUT)
15led.off()
16d = dht.DHT22(machine.Pin(23))
17
18# ************************
19# Configure the ESP32 wifi
20# as STAtion mode.
21import network
22import wifi_credentials
23
24sta = network.WLAN(network.STA_IF)
25if not sta.isconnected():
26 print('connecting to network...')
27 sta.active(True)
28 #sta.connect('your wifi ssid', 'your wifi password')
29 sta.connect(wifi_credentials.ssid, wifi_credentials.password)
30 while not sta.isconnected():
31 pass
32 print('network config:', sta.ifconfig())
33
34# ************************
35# Configure the socket connection
36# over TCP/IP
37import socket
38
39# AF_INET - use Internet Protocol v4 addresses
40# SOCK_STREAM means that it is a TCP socket.
41# SOCK_DGRAM means that it is a UDP socket.
42s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
43s.bind(('',80)) # specifies that the socket is reachable by any address the machine happens to have
44s.listen(5) # max of 5 socket connections
45
46# ************************
47# Function for creating the
48# web page to be displayed
49def web_page():
50 html_page = """
51
52 <html>
53 <head>
54 <meta name='viewport' content='width=device-width, initial-scale=1.0'/>
55 <script>
56 var ajaxRequest = new XMLHttpRequest();
57
58 function ajaxLoad(ajaxURL)
59 {
60 ajaxRequest.open('GET',ajaxURL,true);
61 ajaxRequest.onreadystatechange = function()
62 {
63 if(ajaxRequest.readyState == 4 && ajaxRequest.status==200)
64 {
65 var ajaxResult = ajaxRequest.responseText;
66 var tmpArray = ajaxResult.split("|");
67 document.getElementById('temp').innerHTML = tmpArray[0];
68 document.getElementById('humi').innerHTML = tmpArray[1];
69 }
70 }
71 ajaxRequest.send();
72 }
73
74 function updateDHT()
75 {
76 ajaxLoad('getDHT');
77 }
78
79 setInterval(updateDHT, 3000);
80
81 </script>
82
83
84 <title>ESP32 Weather Station</title>
85 </head>
86
87 <body>
88 <center>
89 <div id='main'>
90 <h1>MicroPython Weather Station</h1>
91 <h4>Web server on ESP32 | DHT values auto updates using AJAX.</h4>
92 <div id='content'>
93 <p>Temperature: <strong><span id='temp'>--.-</span> °C</strong></p>
94 <p>Humidity: <strong><span id='humi'>--.-</span> % </strong></p>
95 </div>
96 </div>
97 </center>
98 </body>
99 </html>
100"""
101 return html_page
102
103while True:
104
105 # Socket accept()
106 conn, addr = s.accept()
107 print("Got connection from %s" % str(addr))
108
109 # Socket receive()
110 request=conn.recv(1024)
111 print("")
112 print("Content %s" % str(request))
113
114 # Socket send()
115 request = str(request)
116 update = request.find('/getDHT')
117 if update == 6:
118 d.measure()
119 t = d.temperature()
120 h = d.humidity()
121 response = str(t) + "|"+ str(h)
122 led.value(not led.value())
123 else:
124 response = web_page()
125
126 # Create a socket reply
127 conn.send('HTTP/1.1 200 OK\n')
128 conn.send('Content-Type: text/html\n')
129 conn.send('Connection: close\n\n')
130 conn.sendall(response)
131
132 # Socket close()
133 conn.close()
References And Credits
-
W3Schools Ajax Introduction:
https://www.w3schools.com/js/js_ajax_intro.asp -
W3Schools setInterval Method:
https://www.w3schools.com/jsref/met_win_setinterval.asp
Posts in this series
- 026 - ESP32 MicroPython: MFRC522 RFID Module
- 025 - ESP32 MicroPython: ESP32 Bluetooth Low Energy
- 024 - ESP32 MicroPython: How to Use SD Card in MicroPython
- 023 - ESP32 MicroPython: Binary Clock
- 022 - ESP32 MicroPython: MQTT Part 2: Subscribe
- 021 - ESP32 MicroPython: MQTT Part 1: Publish
- 020 - ESP32 MicroPython: RESTful APIs | Demo READ and WRITE
- 019 - ESP32 MicroPython: OpenWeather | RESTful APIs
- 018 - ESP32 MicroPython: Thingspeak | RESTful APIs
- 016 - ESP32 MicroPython: Web Server | ESP32 Access Point
- 015 - ESP32 MicroPython: Web Server | ESP32 Station Mode in MicroPython
- 014 - ESP32 MicroPython: SIM800L GSM Module in MicroPython
- 013 - ESP32 MicroPython: UART Serial in MicroPython
- 012 - ESP32 MicroPython: HC-SR04 Ultrasonic Sensor in MicroPython
- 011 - ESP32 MicroPython: DHT11, DHT22 in MicroPython
- 010 - ESP32 MicroPython: 0.96 OLED in MicroPython
- 009 - ESP32 MicroPython: Non-blocking Delays and Multithreading | Multitasking
- 008 - ESP32 MicroPython: Hardware Timer Interrupts
- 007 - ESP32 MicroPython: How to make some sound with MicroPython
- 006 - ESP32 MicroPython: How to control servo motor with MicroPython
- 005 - ESP32 MicroPython: Pulse Width Modulation
- 004 - ESP32 MicroPython: External Interrupts
- 003 - ESP32 MicroPython: General Purpose Input Output | GPIO Pins
- 001 - ESP32 MicroPython: What is MicroPython
- 000 - ESP32 MicroPython: How to Get Started with MicroPython
1 comment
hildi