007 - ESP32 MicroPython: How to make some sound with MicroPython
Introduction
In this article, we will be exploring the use of Pulse Width Modulation or PWM in producing a sound using MicroPython.
Bill Of Materials
- ESP32 Development board.
- A buzzer module or a speaker.
- Some connecting wires.
Circuit Diagram
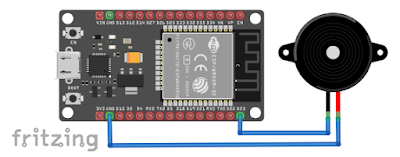
Video Demonstration
Call To Action
If you found this tutorial as helpful, please consider supporting me by Subscribing to my Youtube channel: Click this to Subscribe to TechToTinker
Source Code
Example 1, Single Note:
1# How to make some sound with MicroPython
2# Author: George Bantique,
3# TechToTinker Youtube channel
4# www.techtotinker.com
5# Date: September 18, 2020
6
7# Import the machine module for GPIO and PWM
8import machine
9# Import the time module to add some delays
10import time
11
12# Create a regular GPIO object from pin 23
13p23 = machine.Pin(23, machine.Pin.OUT)
14
15# Create a new object and attach the pwm driver
16buzzer = machine.PWM(p23)
17
18# Set a pwm frequency
19buzzer.freq(1047)
20# Set the pwm duty value
21# this serves as volume control
22# Max volume is a duty value of 512
23buzzer.duty(50)
24# Let the sound ring for a certain duration
25time.sleep(1)
26# Turn off the pulse by setting the duty to 0
27buzzer.duty(0)
28# And disconnect the pwm driver to the GPIO pin
29buzzer.deinit()
py
Example 2, Mario Melody:
1# How to make some sound with MicroPython
2# Author: George Bantique,
3# TechToTinker Youtube channel
4# www.techtotinker.com
5# Date: September 18, 2020
6from machine import Pin
7from machine import PWM
8from time import sleep_ms
9
10class GORILLACELL_BUZZER:
11 def __init__(self, sig_pin):
12 self.pwm = PWM(Pin(sig_pin),duty_u16=0)
13
14 def play(self, melodies, wait, duty=32767):
15 for note in melodies:
16 if note != 0:
17 self.pwm.freq(note)
18 self.pwm.duty_u16(duty)
19 sleep_ms(wait)
20 # Disable the pulse, setting the duty to 0
21 self.pwm.duty_u16(0)
22 # Disconnect the pwm driver
23 #self.pwm.deinit() # remove to play the next melodies
24
25 def tone(self, notes, wait, duty=32767):
26 self.pwm.freq(notes)
27 self.pwm.duty_u16(duty)
28 sleep_ms(wait)
29 self.pwm.duty_u16(0)
30
31# Notes and its equivalent frequency
32 # Octave 0 ********************
33B0 = 31 # B
34 # Octave 1 ********************
35C1 = 33 # C
36CS1 = 35 # C#/Db
37D1 = 37 # D
38DS1 = 39 # D#/Eb
39E1 = 41 # E
40F1 = 44 # F
41FS1 = 46 # F#/Gb
42G1 = 49 # G
43GS1 = 52 # G#/Ab
44A1 = 55 # A
45AS1 = 58 # A#/Bb
46B1 = 62 # B
47 # Octave 2 ********************
48C2 = 65 # C
49CS2 = 69 # C#/Db
50D2 = 73 # D
51DS2 = 78 # D#/Eb
52E2 = 82 # E
53F2 = 87 # F
54FS2 = 93 # F#/Gb
55G2 = 98 # G
56GS2 = 104 # G#/Ab
57A2 = 110 # A
58AS2 = 117 # A#/Bb
59B2 = 123 # B
60 # Octave 3 ********************
61C3 = 131 # C
62CS3 = 139 # C#/Db
63D3 = 147 # D
64DS3 = 156 # D#/Eb
65E3 = 165 # E
66F3 = 175 # F
67FS3 = 185 # F#/Gb
68G3 = 196 # G
69GS3 = 208 # G#/Ab
70A3 = 220 # A
71AS3 = 233 # A#/Bb
72B3 = 247 # B
73 # Octave 4 ********************
74C4 = 262 # C
75CS4 = 277 # C#/Db
76D4 = 294 # D
77DS4 = 311 # D#/Eb
78E4 = 330 # E
79F4 = 349 # F
80FS4 = 370 # F#/Gb
81G4 = 392 # G
82GS4 = 415 # G#/Ab
83A4 = 440 # A
84AS4 = 466 # A#/Bb
85B4 = 494 # B
86 # Octave 5 ********************
87C5 = 523 # C
88CS5 = 554 # C#/Db
89D5 = 587 # D
90DS5 = 622 # D#/Eb
91E5 = 659 # E
92F5 = 698 # F
93FS5 = 740 # F#/Gb
94G5 = 784 # G
95GS5 = 831 # G#/Ab
96A5 = 880 # A
97AS5 = 932 # A#/Bb
98B5 = 988 # B
99 # Octave 6 ********************
100C6 = 1047 # C
101CS6 = 1109 # C#/Db
102D6 = 1175 # D
103DS6 = 1245 # D#/Eb
104E6 = 1319 # E
105F6 = 1397 # F
106FS6 = 1480 # F#/Gb
107G6 = 1568 # G
108GS6 = 1661 # G#/Ab
109A6 = 1760 # A
110AS6 = 1865 # A#/Bb
111B6 = 1976 # B
112 # Octave 7 ********************
113C7 = 2093 # C
114CS7 = 2217 # C#/Db
115D7 = 2349 # D
116DS7 = 2489 # D#/Eb
117E7 = 2637 # E
118F7 = 2794 # F
119FS7 = 2960 # F#/Gb
120G7 = 3136 # G
121GS7 = 3322 # G#/Ab
122A7 = 3520 # A
123AS7 = 3729 # A#/Bb
124B7 = 3951 # B
125 # Octave 8 ********************
126C8 = 4186 # C
127CS8 = 4435 # C#/Db
128D8 = 4699 # D
129DS8 = 4978 # D#/Eb
130
131
132
133# This is the list of notes for mario theme
134# 0 denotes rest notes
135mario = [
136 E7, E7, 0, E7, 0, C7, E7, 0,
137 G7, 0, 0, 0, G6, 0, 0, 0,
138 C7, 0, 0, G6, 0, 0, E6, 0,
139 0, A6, 0, B6, 0,AS6, A6, 0,
140 G6, E7, 0, G7, A7, 0, F7, G7,
141 0, E7, 0, C7, D7, B6, 0, 0,
142 C7, 0, 0, G6, 0, 0, E6, 0,
143 0, A6, 0, B6, 0,AS6, A6, 0,
144 G6, E7, 0, G7, A7, 0, F7, G7,
145 0, E7, 0, C7, D7, B6, 0, 0,
146 ]
147
148# This is the list of notes for jingle bells
149jingle = [
150 E7, E7, E7, 0,
151 E7, E7, E7, 0,
152 E7, G7, C7, D7, E7, 0,
153 F7, F7, F7, F7, F7, E7, E7, E7, E7, D7, D7, E7, D7, 0, G7, 0,
154 E7, E7, E7, 0,
155 E7, E7, E7, 0,
156 E7, G7, C7, D7, E7, 0,
157 F7, F7, F7, F7, F7, E7, E7, E7, G7, G7, F7, D7, C7, 0
158 ]
159
160# This is the list of notes for Twinkle, Twinkle Little Star
161twinkle = [
162 C6, C6, G6, G6, A6, A6, G6, 0,
163 F6, F6, E6, E6, D6, D6, C6, 0,
164 G6, G6, F6, F6, E6, E6, D6, 0,
165 G6, G6, F6, F6, E6, E6, D6, 0,
166 C6, C6, G6, G6, A6, A6, G6, 0,
167 F6, F6, E6, E6, D6, D6, C6, 0,
168 ]
169
170
171buzzer = GORILLACELL_BUZZER(10)
172
173print("Playing mario.")
174buzzer.play(mario, 150, 32767)
175sleep_ms(1000)
176
177print("Playing jingle bells.")
178buzzer.play(jingle, 250, 32767)
179sleep_ms(1000)
180
181print("Playing twinkle, twinkle little star.")
182buzzer.play(twinkle, 600, 32767)
py
Example 3, More Melodies:
1# How to make some sound with MicroPython
2# Author: George Bantique,
3# TechToTinker Youtube channel
4# www.techtotinker.com
5# Date: September 18, 2020
6
7# This is the list of notes for jingle bells
8jingle = [
9 E7, E7, E7, 0,
10 E7, E7, E7, 0,
11 E7, G7, C7, D7, E7, 0,
12 F7, F7, F7, F7, F7, E7, E7, E7, E7, D7, D7, E7, D7, 0, G7, 0,
13 E7, E7, E7, 0,
14 E7, E7, E7, 0,
15 E7, G7, C7, D7, E7, 0,
16 F7, F7, F7, F7, F7, E7, E7, E7, G7, G7, F7, D7, C7, 0
17]
18
19def play_jingle():
20 play(p23, jingle, 0.25, 512)
21
22# This is the list of notes for Twinkle, Twinkle Little Star
23twinkle = [
24 C6, C6, G6, G6, A6, A6, G6, 0,
25 F6, F6, E6, E6, D6, D6, C6, 0,
26 G6, G6, F6, F6, E6, E6, D6, 0,
27 G6, G6, F6, F6, E6, E6, D6, 0,
28 C6, C6, G6, G6, A6, A6, G6, 0,
29 F6, F6, E6, E6, D6, D6, C6, 0,
30]
31
32def play_twinkle():
33 play(p23, twinkle, 0.6, 50)
py
Posts in this series
- 026 - ESP32 MicroPython: MFRC522 RFID Module
- 025 - ESP32 MicroPython: ESP32 Bluetooth Low Energy
- 024 - ESP32 MicroPython: How to Use SD Card in MicroPython
- 023 - ESP32 MicroPython: Binary Clock
- 022 - ESP32 MicroPython: MQTT Part 2: Subscribe
- 021 - ESP32 MicroPython: MQTT Part 1: Publish
- 020 - ESP32 MicroPython: RESTful APIs | Demo READ and WRITE
- 019 - ESP32 MicroPython: OpenWeather | RESTful APIs
- 018 - ESP32 MicroPython: Thingspeak | RESTful APIs
- 017 - ESP32 MicroPython: DHT Values Auto Updates using AJAX
- 016 - ESP32 MicroPython: Web Server | ESP32 Access Point
- 015 - ESP32 MicroPython: Web Server | ESP32 Station Mode in MicroPython
- 014 - ESP32 MicroPython: SIM800L GSM Module in MicroPython
- 013 - ESP32 MicroPython: UART Serial in MicroPython
- 012 - ESP32 MicroPython: HC-SR04 Ultrasonic Sensor in MicroPython
- 011 - ESP32 MicroPython: DHT11, DHT22 in MicroPython
- 010 - ESP32 MicroPython: 0.96 OLED in MicroPython
- 009 - ESP32 MicroPython: Non-blocking Delays and Multithreading | Multitasking
- 008 - ESP32 MicroPython: Hardware Timer Interrupts
- 006 - ESP32 MicroPython: How to control servo motor with MicroPython
- 005 - ESP32 MicroPython: Pulse Width Modulation
- 004 - ESP32 MicroPython: External Interrupts
- 003 - ESP32 MicroPython: General Purpose Input Output | GPIO Pins
- 001 - ESP32 MicroPython: What is MicroPython
- 000 - ESP32 MicroPython: How to Get Started with MicroPython
No comments yet!