009 - ESP32 MicroPython: Non-blocking Delays and Multithreading | Multitasking
Introduction
In previous tutorials we learned to use the hardware timer interrupt which is very useful when we need to execute a task at specific interval period. We also use hardware timer interrupts for executing timed threaded task which is similar to multitasking. Using the hardware timer in that manner, sooner or later, we will runout of resources because ESP32 have 4 hardware timer only namely Timer0 to Timer3.
In this video, we will try to explore alternative ways of doing multiple task at almost the same time using the time module.
Circuit Diagram
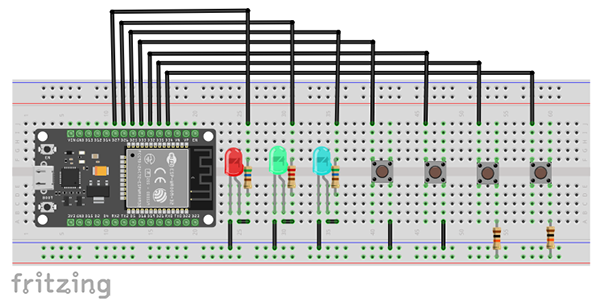
Hardware Instruction
- Connect the ESP32 development board to the breadboard.
- Connect the cathode lead of the red LED to the ground while the anode pin through limiting resistor to GPIO D27 of ESP32.
- Connect the cathode lead of the green LED to the ground while the anode pin through limiting resistor to GPIO D26 of ESP32.
- Connect the cathode lead of the blue LED to the ground while the anode pin through limiting resistor to GPIO D25 of ESP32.
- Connect the ‘mode’ tactile switch with one pin to the ground while the other to GPIO D33 of ESP32.
- Connect the ‘left’ tactile switch with one pin to the ground while the other to GPIO D32 of ESP32.
- Connect the ‘rght’ tactile switch with one pin to the ground through a 10K resistor while the other to GPIO D35 of ESP32.
- Connect the ‘entr’ tactile switch with one pin to the ground through a 10K resistor while the other to GPIO D34 of ESP32.
Pins D32 and D33 of ESP32 has internal pull-up resistor so take advantage of it while for pins D34 and D35 the internal pull-ups is NOT PRESENT so we use external pull-up resistor of 10 kilo Ohms which works exactly the same. The button switches are configured as ACTIVE-LOW, meaning the value when press is LOW or 0.
Video Demonstration
Call To Action
If you have any question regarding this tutorial, please do not hesitate to write it in the comment box provided.
You may also like to Subscribe to my Youtube Channel. Please click this to Subscribe to TechToTinker Youtube Channel.
Thank you and have a good days ahead.
Source Code
Example # 1 Blinking an LED, Blocking Code:
1
2import machine
3import time
4
5led = machine.Pin(2, machine.Pin.OUT)
6
7while True:
8<span> </span>led.on()
9 time.sleep(0.5)
10 led.off()
11 time.sleep(0.5)
Example # 2 Blinking an LED, Non-Blocking Code:
1
2import machine
3import time
4
5led = machine.Pin(2, machine.Pin.OUT)
6
7start_time = time.ticks_ms()
8interval = 500 # 500 ms interval
9led_state = 0
10
11while True:
12 if ( time.ticks_ms() - start_time > interval ):
13 if led_state == 1:
14 led_state = 0
15 else:
16 led_state = 1
17 led.value(led_state)
18 start_time = time.ticks_ms()
Example # 3 Multi-threading or doing multiple task at almost the same time:
1
2import machine
3import time
4
5red = machine.Pin(27, machine.Pin.OUT)
6grn = machine.Pin(26, machine.Pin.OUT)
7blu = machine.Pin(25, machine.Pin.OUT)
8
9mode = machine.Pin(33, machine.Pin.IN, machine.Pin.PULL_UP)
10left = machine.Pin(32, machine.Pin.IN, machine.Pin.PULL_UP)
11rght = machine.Pin(35, machine.Pin.IN)
12entr = machine.Pin(34, machine.Pin.IN)
13
14r_start = time.ticks_ms()
15g_start = time.ticks_ms()
16b_start = time.ticks_ms()
17k_start = time.ticks_ms()
18
19r_interval = 300
20g_interval = 500
21b_interval = 700
22k_interval = 200
23
24state = 0
25EDIT_RESOLUTION = 10
26reset = 0
27
28print('**************************')
29print(' DEFAULT Interval Values ')
30print('--------------------------')
31print('Red interval:', r_interval, 'ms')
32print('Grn interval:', g_interval, 'ms')
33print('Blu interval:', b_interval, 'ms')
34print('**************************')
35
36while True:
37 if time.ticks_ms() - r_start >= r_interval:
38 red.value( not red.value() )
39 r_start = time.ticks_ms()
40 if time.ticks_ms() - g_start >= g_interval:
41 grn.value( not grn.value() )
42 g_start = time.ticks_ms()
43 if time.ticks_ms() - b_start >= b_interval:
44 blu.value( not blu.value() )
45 b_start = time.ticks_ms()
46 if time.ticks_ms() - k_start >= k_interval:
47 k_start = time.ticks_ms()
48
49 if mode.value()==0:
50 if state==0: # idle mode
51 state = 1
52 print()
53 print('*************')
54 print('Red edit mode')
55 print('-------------')
56 elif state==1: # red edit mode
57 state = 2
58 print()
59 print('*************')
60 print('Grn edit mode')
61 print('-------------')
62 elif state==2: # grn edit mode
63 state = 3
64 print()
65 print('*************')
66 print('Blu edit mode')
67 print('-------------')
68 elif state==3: # blu edit mode
69 state = 0
70 print()
71 print('*************')
72 print('Idle mode')
73 print('-------------')
74
75 if left.value()==0:
76 if state==1: # red edit mode
77 if r_interval - EDIT_RESOLUTION > 0:
78 r_interval -= EDIT_RESOLUTION
79 print('Red interval:', r_interval, 'ms')
80 elif state==2: # grn edit mode
81 if g_interval - EDIT_RESOLUTION > 0:
82 g_interval -= EDIT_RESOLUTION
83 print('Grn interval:', g_interval, 'ms')
84 elif state==3: # blu edit mode
85 if b_interval - EDIT_RESOLUTION > 0:
86 b_interval -= EDIT_RESOLUTION
87 print('Blu interval:', b_interval, 'ms')
88
89 if rght.value()==0:
90 if state==1: # red edit mode
91 r_interval += EDIT_RESOLUTION
92 print('Red interval:', r_interval, 'ms')
93 elif state==2: # grn edit mode
94 g_interval += EDIT_RESOLUTION
95 print('Grn interval:', g_interval, 'ms')
96 elif state==3: # blu edit mode
97 b_interval += EDIT_RESOLUTION
98 print('Blu interval:', b_interval, 'ms')
99
100 if entr.value()==0:
101 r_interval = 300
102 g_interval = 500
103 b_interval = 700
104 print()
105 print('**************************')
106 print('Values RESETTED to DEFAULT')
107 print('--------------------------')
108 print('Red interval:', r_interval, 'ms')
109 print('Grn interval:', g_interval, 'ms')
110 print('Blu interval:', b_interval, 'ms')
111 print('**************************')
Posts in this series
- 026 - ESP32 MicroPython: MFRC522 RFID Module
- 025 - ESP32 MicroPython: ESP32 Bluetooth Low Energy
- 024 - ESP32 MicroPython: How to Use SD Card in MicroPython
- 023 - ESP32 MicroPython: Binary Clock
- 022 - ESP32 MicroPython: MQTT Part 2: Subscribe
- 021 - ESP32 MicroPython: MQTT Part 1: Publish
- 020 - ESP32 MicroPython: RESTful APIs | Demo READ and WRITE
- 019 - ESP32 MicroPython: OpenWeather | RESTful APIs
- 018 - ESP32 MicroPython: Thingspeak | RESTful APIs
- 017 - ESP32 MicroPython: DHT Values Auto Updates using AJAX
- 016 - ESP32 MicroPython: Web Server | ESP32 Access Point
- 015 - ESP32 MicroPython: Web Server | ESP32 Station Mode in MicroPython
- 014 - ESP32 MicroPython: SIM800L GSM Module in MicroPython
- 013 - ESP32 MicroPython: UART Serial in MicroPython
- 012 - ESP32 MicroPython: HC-SR04 Ultrasonic Sensor in MicroPython
- 011 - ESP32 MicroPython: DHT11, DHT22 in MicroPython
- 010 - ESP32 MicroPython: 0.96 OLED in MicroPython
- 008 - ESP32 MicroPython: Hardware Timer Interrupts
- 007 - ESP32 MicroPython: How to make some sound with MicroPython
- 006 - ESP32 MicroPython: How to control servo motor with MicroPython
- 005 - ESP32 MicroPython: Pulse Width Modulation
- 004 - ESP32 MicroPython: External Interrupts
- 003 - ESP32 MicroPython: General Purpose Input Output | GPIO Pins
- 001 - ESP32 MicroPython: What is MicroPython
- 000 - ESP32 MicroPython: How to Get Started with MicroPython
No comments yet!