022 - MicroPython TechNotes: Potentiometer | Reading an Analog Input
Introduction
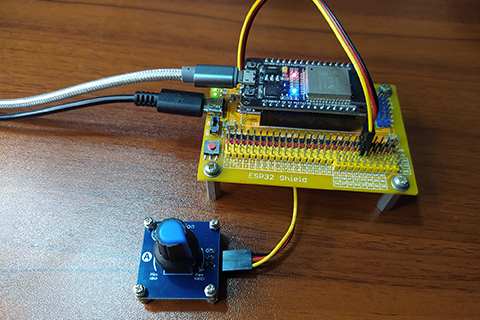
In this article, we will learn how to use potentiometer with ESP32 using MicroPython programming langauge.
Pinout
- G – for the ground.
- V – for the supply voltage.
- S – for the signal pin.
Bill Of Materials
- ESP32 development board that will serve as the brain of this experiment.
- ESP32 shield from Gorillacell ESP32 development kit to extend ESP32 pins to pin headers for easy circuit connection.
- 3-pin female-female dupont wires to attach the potentiometer module to ESP32 shield.
- Potentiometer module.
Hardware Instruction
- First, attach the ESP32 board on top of the ESP32 shield making sure that all pins are properly aligned and both USB port are on the same side.
- Next, attach the dupont wires to potentiometer module by following the color coding which is black for the ground, red for the VCC, and yellow for the signal pin.
- Next, attach the other end of the dupont wires to the ESP32 shield by matching the colors of the wires to the colors of the pin headers which is black to black, red to red, and yellow to yellow. For this, I choose GPIO 32 to serve as the analog input pin from the potentiometer.
- Next, power the ESP32 shield with an external power supply with a type-C USB connector cable and make sure that the power switch is set to ON state.
- Next, connect the ESP32 shield to the computer through a micro-USB cable. The demo circuit should now be ready.
Software Instruction
I prepared 2 example source code for this experiment. Copy each one-by-one.
Modify it according to what you want.
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example # 1, basics of reading an analog input:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC
5
6p32 = Pin(32, Pin.IN)
7pot = ADC(p32)
8pot.atten(ADC.ATTN_11DB)
9
10# The following line of code should be tested in the REPL:
11# This will read the analog values of pot object and
12# print it to the REPL
13print(pot.read())
py
2. Example # 2, simple application in using a potentiometer module:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC, PWM
5from time import sleep_ms
6
7p32 = Pin(32, Pin.IN)
8pot = ADC(p32)
9pot.atten(ADC.ATTN_11DB)
10
11p2 = Pin(2, Pin.OUT)
12led = PWM(p2)
13led.freq(60)
14
15def map(x, in_min, in_max, out_min, out_max):
16 return int((x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min)
17
18while True:
19 pot_value = pot.read() # 0 - 4095
20 pwm_value = map(pot_value, 0, 4095, 0, 1023)
21 led.duty(pwm_value) # 0 - 1023
22 sleep_ms(500)
py
References And Credits
-
Purchase your Gorillacell ESP32 development kit from: gorillacell.kr
-
ESP32 Quick Reference: https://docs.micropython.org/en/latest/esp32/quickref.html
Posts in this series
- 049 - MicroPython TechNotes: MP3 Player
- 048 - MicroPython TechNotes: Analog Touch Sensor
- 047 - MicroPython TechNotes: E108 GPS
- 046 - MicroPython TechNotes: RF433 Transceivers
- 045 - MicroPython TechNotes: Infrared Transmitter
- 044 - MicroPython TechNotes: Infrared Receiver
- 043 - MicroPython TechNotes: ESP12E WiFi | External WiFi module
- 042 - MicroPython TechNotes: JDY-32 | Bluetooth Low Energy BLE
- 041 - MicroPython TechNotes: Bluetooth HC-06
- 040 - MicroPython TechNotes: Relay
- 039 - MicroPython TechNotes: Electromagnet
- 038 - MicroPython TechNotes: Buzzer
- 037 - MicroPython TechNotes: Servo Motor
- 036 - MicroPython TechNotes: Stepper Motor
- 035 - MicroPython TechNotes: Dual Motor Driver
- 034 - MicroPython TechNotes: DC Motors | Gear Motor and Fan Motor
- 033 - MicroPython TechNotes: TCS34725 RGB Color Sensor
- 032 - MicroPython TechNotes: BMP280 Sensor
- 031 - MicroPython TechNotes: TOF Distance Sensor
- 030 - MicroPython TechNotes: DS3231 RTC
- 029 - MicroPython TechNotes: HC-SR04 Ultrasonic Sensor
- 028 - MicroPython TechNotes: DHT11 Temperature and Humidity Sensor
- 027 - MicroPython TechNotes: Rotary Encoder
- 026 - MicroPython TechNotes: Light Dependent Resistor (LDR)
- 025 - MicroPython TechNotes: Joystick
- 024 - MicroPython TechNotes: Slider Switch
- 023 - MicroPython TechNotes: Continuous Rotation Potentiometer
- 021 - MicroPython TechNotes: Color Touch Sensor
- 020 - MicroPython TechNotes: Touch Sensor
- 019 - MicroPython TechNotes: Switch Module
- 018 - MicroPython TechNotes: Button | Reading an Input
- 017 - MicroPython TechNotes: LASER Module
- 016 - MicroPython TechNotes: RGB LED Matrix
- 015 - MicroPython TechNotes: Neopixel 16
- 014 - MicroPython TechNotes: 8x8 Dot Matrix Display (I2C)
- 013 - MicroPython TechNotes: 8x16 Dot Matrix Display (SPI)
- 012 - MicroPython TechNotes: 8x8 Dot Matrix Display (SPI)
- 011 - MicroPython TechNotes: 1.3 OLED Display
- 010 - MicroPython TechNotes: 0.96 OLED Display
- 009 - MicroPython TechNotes: 7 Segment Display
- 008 - MicroPython TechNotes: 16x2 LCD
- 007 - MicroPython TechNotes: RGB LED
- 006 - MicroPython TechNotes: Traffic Light LED Module
- 005 - MicroPython TechNotes: Gorilla Cell LED | MicroPython Hello World
- 004 - MicroPython TechNotes: Gorilla Cell I/O Devices
- 003 - MicroPython TechNotes: Gorillacell ESP32 Shield
- 002 - MicroPython TechNotes: Introduction for Gorillacell ESP32 Dev Kit
- 001 - MicroPython TechNotes: Get Started with MicroPython
- 000 - MicroPython TechNotes: Unboxing Gorillacell ESP32 Development Kit
No comments yet!