041 - MicroPython TechNotes: Bluetooth HC-06
Introduction
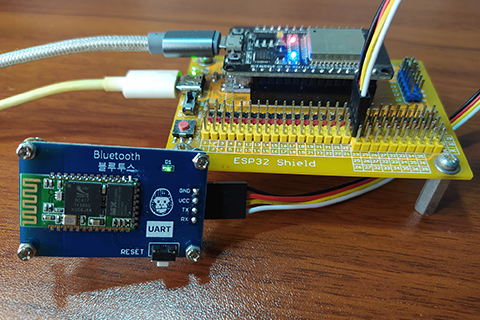
In this article, I will talk about the (external) BLUETOOTH module with ESP32 using MicroPython.
Bill Of Materials
To follow this lesson you will need the following:
- ESP32 development board.
- Gorillacell ESP32 shield.
- 4-pin female-female dupont wires.
- Gorillacell Bluetooth module.

Pinout
- GND – for the ground pin.
- VCC – for the supply voltage.
- TX – for the UART transmit pin.
- RX – for the UART receive pin.
Hardware Instruction
- Connect the Bluetooth module GND pin to ESP32 GND pin.
- Connect the Bluetooth module VCC pin to ESP32 3.3V pin.
- Connect the Bluetooth module TX pin to ESP32 GPIO 23 pin.
- Connect the Bluetooth module RX pin to ESP32 GPIO 25 pin.
Software Instruction
- Copy the sample source code below and paste it to your Thonny IDE.
- Run it then modify according to your needs.
- Enjoy and happy learning.
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example # 1, exploring the basics:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3from machine import UART
4bt = UART(2, baudrate=9600, tx=25, rx=23)
5
6# The following lines of code can be tested using the REPL:
7# 1. To check if there is available serial data<br></br># bt.any()
8# 2. To read all data available:<br></br>
9# bt.read()<br></br>
10# 3. Or you can input the number of bytes as parameter
11# you want to read from uart
12# bt.read(num_here)
13# 4. Or you can read 1 line
14# bt.readline()
15# 5. Now to write / transmit uart data
16# bt.write(your_data)<br></br>
py
2. Example # 2, controlling an output:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3from machine import Pin
4from machine import UART
5bt = UART(2, baudrate=9600, tx=25, rx=23)
6led = Pin(2, Pin.OUT)
7while True:
8 if bt.any()!=0:
9 msg = bt.read(bt.any()).decode().strip('rn')
10 print(msg)
11 if "ON" in msg:
12 led.value(1)
13 if "OFF" in msg:
14 led.value(0)
py
3. Example # 3, reading an input:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3from machine import Pin
4from machine import UART
5from machine import Timer
6from time import ticks_ms
7
8bt = UART(2, baudrate=9600, tx=25, rx=23)
9led = Pin(2, Pin.OUT)
10sw = Pin(0, Pin.IN)
11
12tim0 = Timer(0)
13t_start = ticks_ms()
14
15while True:
16 if bt.any()!=0:
17 #msg = bt.read(bt.any()).decode().strip('rn')
18 #print(msg)
19 msg = bt.read(bt.any()).decode()
20 if "ON" in msg:
21 led.value(1)
22 tim0.deinit()
23 print('LED is ON')
24 elif "OFF" in msg:
25 led.value(0)
26 tim0.deinit()
27 print('LED is OFF')
28 elif "BLINK" in msg:
29 tim0.init(period=250, mode=Timer.PERIODIC, callback=lambda t: led.value(not led.value()))
30 print('LED is blinking')
31 else:
32 print(msg.strip('rn'))
33 if ticks_ms()-t_start >= 300:
34 if sw.value()==0: # BOOT button is pressed
35 bt.write('Boot button is pressed.rn')
36 print('Sending "Boot button is pressed."')
37 t_start=ticks_ms()
py
References And Credits
- Purchased your Gorillacell ESP32 development kit at: https://gorillacella.kr/
Posts in this series
- 049 - MicroPython TechNotes: MP3 Player
- 048 - MicroPython TechNotes: Analog Touch Sensor
- 047 - MicroPython TechNotes: E108 GPS
- 046 - MicroPython TechNotes: RF433 Transceivers
- 045 - MicroPython TechNotes: Infrared Transmitter
- 044 - MicroPython TechNotes: Infrared Receiver
- 043 - MicroPython TechNotes: ESP12E WiFi | External WiFi module
- 042 - MicroPython TechNotes: JDY-32 | Bluetooth Low Energy BLE
- 040 - MicroPython TechNotes: Relay
- 039 - MicroPython TechNotes: Electromagnet
- 038 - MicroPython TechNotes: Buzzer
- 037 - MicroPython TechNotes: Servo Motor
- 036 - MicroPython TechNotes: Stepper Motor
- 035 - MicroPython TechNotes: Dual Motor Driver
- 034 - MicroPython TechNotes: DC Motors | Gear Motor and Fan Motor
- 033 - MicroPython TechNotes: TCS34725 RGB Color Sensor
- 032 - MicroPython TechNotes: BMP280 Sensor
- 031 - MicroPython TechNotes: TOF Distance Sensor
- 030 - MicroPython TechNotes: DS3231 RTC
- 029 - MicroPython TechNotes: HC-SR04 Ultrasonic Sensor
- 028 - MicroPython TechNotes: DHT11 Temperature and Humidity Sensor
- 027 - MicroPython TechNotes: Rotary Encoder
- 026 - MicroPython TechNotes: Light Dependent Resistor (LDR)
- 025 - MicroPython TechNotes: Joystick
- 024 - MicroPython TechNotes: Slider Switch
- 023 - MicroPython TechNotes: Continuous Rotation Potentiometer
- 022 - MicroPython TechNotes: Potentiometer | Reading an Analog Input
- 021 - MicroPython TechNotes: Color Touch Sensor
- 020 - MicroPython TechNotes: Touch Sensor
- 019 - MicroPython TechNotes: Switch Module
- 018 - MicroPython TechNotes: Button | Reading an Input
- 017 - MicroPython TechNotes: LASER Module
- 016 - MicroPython TechNotes: RGB LED Matrix
- 015 - MicroPython TechNotes: Neopixel 16
- 014 - MicroPython TechNotes: 8x8 Dot Matrix Display (I2C)
- 013 - MicroPython TechNotes: 8x16 Dot Matrix Display (SPI)
- 012 - MicroPython TechNotes: 8x8 Dot Matrix Display (SPI)
- 011 - MicroPython TechNotes: 1.3 OLED Display
- 010 - MicroPython TechNotes: 0.96 OLED Display
- 009 - MicroPython TechNotes: 7 Segment Display
- 008 - MicroPython TechNotes: 16x2 LCD
- 007 - MicroPython TechNotes: RGB LED
- 006 - MicroPython TechNotes: Traffic Light LED Module
- 005 - MicroPython TechNotes: Gorilla Cell LED | MicroPython Hello World
- 004 - MicroPython TechNotes: Gorilla Cell I/O Devices
- 003 - MicroPython TechNotes: Gorillacell ESP32 Shield
- 002 - MicroPython TechNotes: Introduction for Gorillacell ESP32 Dev Kit
- 001 - MicroPython TechNotes: Get Started with MicroPython
- 000 - MicroPython TechNotes: Unboxing Gorillacell ESP32 Development Kit
No comments yet!