025 - MicroPython TechNotes: Joystick
Introduction
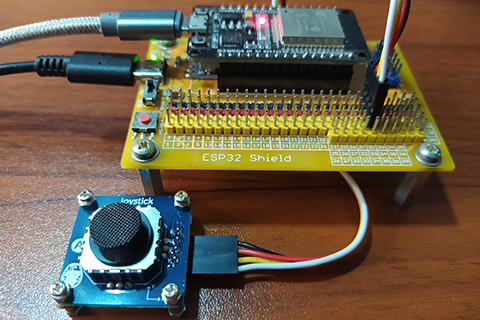
In this article, we will tackle how to use JOYSTICK with ESP32 using MicroPython programming language.
Pinout
- G – for the ground pin.
- V – for the supply voltage.
- x – for the horizontal potentiometer pin.
- y – for the vertical potentiometer pin.
Bill Of Materials
- ESP32 development board to serve as the brain for the experiment.
- ESP32 shield from Gorillacell ESP32 development kit to extend the ESP32 pins to pin headers for easy circuit connection.
- 4-pin female-female dupont jumper wires to connect the joystick module to the ESP32 shield.
- Joystick module itself.
Hardware Instruction
- First, attach the ESP32 development board on top of the ESP32 shield and make sure that both USB ports are on the same side.
- Next, attach the dupont wires to the joystick module by following the color coding which is black for the ground, red for the VCC, yellow for the x pin, and white for the y pin.
- Next, attach the other end of the dupont wires to the ESP32 shield by matching the colors of the wires to the colors of the pin headers which is black to black, red to red, yellow and the following colors to the yellow pin headers.
- Next, power the ESP32 shield with an external power supply with a type-C USB connector and make sure that the power switch is set to ON state.
- Lastly, connect the ESP32 to the computer through a micro-USB cable. Our demo circuit should be now ready.
Software Instruction
I prepared 3 example source code for this demo.
Copy and paste it to your Thonny IDE.
Play with it, modify and adapt according to your needs.
Enjoy and happy tinkering.
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example # 1, basics of reading an analog input just like the joystick module:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC
5from time import sleep_ms
6
7x = ADC(Pin(32, Pin.IN))
8y = ADC(Pin(33, Pin.IN))
9x.atten(ADC.ATTN_11DB)
10y.atten(ADC.ATTN_11DB)
11
12while True:
13 x_val = x.read()
14 y_val = y.read()
15 print('Current position:{},{}'.format(x_val,y_val))
16 sleep_ms(300)
py
2. Example # 2, display joystick position using RGB matrix:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC
5from neopixel import NeoPixel
6from time import sleep_ms
7
8class RGB_Matrix:
9
10 def __init__(self, gpio, width, height):
11 self.width = width
12 self.height = height
13 self.neopixel = NeoPixel(Pin(gpio), width*height)
14
15 def pixel_set(self, row, col, r=0, g=0, b=0):
16 self.neopixel[row + (col*self.width)] = (r, g, b)
17 self.neopixel.write()
18
19 def pixel_clr(self, row, col):
20 self.neopixel[row + (col*self.width)] = (0, 0, 0)
21 self.neopixel.write()
22
23 def clear_all(self):
24 self.neopixel.fill((0,0,0))
25 self.neopixel.write()
26
27
28np = RGB_Matrix(25, 8, 8)
29x = ADC(Pin(32, Pin.IN))
30y = ADC(Pin(33, Pin.IN))
31x.atten(ADC.ATTN_11DB)
32y.atten(ADC.ATTN_11DB)
33
34def map(x, in_min, in_max, out_min, out_max):
35 # This will not handle x value greater than in_max or
36 # x value less than in_min
37 return int((x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min)
38
39while True:
40 x_pos = map(x.read(), 0, 4095, 0, 7)
41 y_pos = map(y.read(), 0, 4095, 7, 0)
42 np.clear_all()
43 np.pixel_set(x_pos, y_pos, 10,0,0)
44 sleep_ms(300)
py
3. Example # 3, control a pixel in RGB matrix using the joystick module:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC
5from neopixel import NeoPixel
6from time import sleep_ms
7
8class RGB_Matrix:
9
10 def __init__(self, gpio, width, height):
11 self.width = width
12 self.height = height
13 self.neopixel = NeoPixel(Pin(gpio), width*height)
14
15 def pixel_set(self, row, col, r=0, g=0, b=0):
16 self.neopixel[row + (col*self.width)] = (r, g, b)
17 self.neopixel.write()
18
19 def pixel_clr(self, row, col):
20 self.neopixel[row + (col*self.width)] = (0, 0, 0)
21 self.neopixel.write()
22
23 def clear_all(self):
24 self.neopixel.fill((0,0,0))
25 self.neopixel.write()
26
27
28np = RGB_Matrix(25, 8, 8)
29x = ADC(Pin(32, Pin.IN))
30y = ADC(Pin(33, Pin.IN))
31x.atten(ADC.ATTN_11DB)
32y.atten(ADC.ATTN_11DB)
33
34curr_x = 3
35curr_y = 3
36
37while True:
38 x_val = x.read()
39 y_val = y.read()
40
41 if x_val < 1850:
42 if curr_x > 0:
43 curr_x = curr_x - 1
44 elif x_val > 1930:
45 if curr_x < 7:
46 curr_x = curr_x + 1
47 if y_val < 1890:
48 if curr_y < 7:
49 curr_y = curr_y + 1
50 elif y_val > 1970:
51 if curr_y > 0:
52 curr_y = curr_y - 1
53 print(curr_x, curr_y)
54
55 np.clear_all()
56 np.pixel_set(curr_x, curr_y, 10, 0, 0)
57 sleep_ms(100)
py
References And Credits
- Purchase your Gorillacell ESP32 development kit at: gorillacell.kr
Posts in this series
- 049 - MicroPython TechNotes: MP3 Player
- 048 - MicroPython TechNotes: Analog Touch Sensor
- 047 - MicroPython TechNotes: E108 GPS
- 046 - MicroPython TechNotes: RF433 Transceivers
- 045 - MicroPython TechNotes: Infrared Transmitter
- 044 - MicroPython TechNotes: Infrared Receiver
- 043 - MicroPython TechNotes: ESP12E WiFi | External WiFi module
- 042 - MicroPython TechNotes: JDY-32 | Bluetooth Low Energy BLE
- 041 - MicroPython TechNotes: Bluetooth HC-06
- 040 - MicroPython TechNotes: Relay
- 039 - MicroPython TechNotes: Electromagnet
- 038 - MicroPython TechNotes: Buzzer
- 037 - MicroPython TechNotes: Servo Motor
- 036 - MicroPython TechNotes: Stepper Motor
- 035 - MicroPython TechNotes: Dual Motor Driver
- 034 - MicroPython TechNotes: DC Motors | Gear Motor and Fan Motor
- 033 - MicroPython TechNotes: TCS34725 RGB Color Sensor
- 032 - MicroPython TechNotes: BMP280 Sensor
- 031 - MicroPython TechNotes: TOF Distance Sensor
- 030 - MicroPython TechNotes: DS3231 RTC
- 029 - MicroPython TechNotes: HC-SR04 Ultrasonic Sensor
- 028 - MicroPython TechNotes: DHT11 Temperature and Humidity Sensor
- 027 - MicroPython TechNotes: Rotary Encoder
- 026 - MicroPython TechNotes: Light Dependent Resistor (LDR)
- 024 - MicroPython TechNotes: Slider Switch
- 023 - MicroPython TechNotes: Continuous Rotation Potentiometer
- 022 - MicroPython TechNotes: Potentiometer | Reading an Analog Input
- 021 - MicroPython TechNotes: Color Touch Sensor
- 020 - MicroPython TechNotes: Touch Sensor
- 019 - MicroPython TechNotes: Switch Module
- 018 - MicroPython TechNotes: Button | Reading an Input
- 017 - MicroPython TechNotes: LASER Module
- 016 - MicroPython TechNotes: RGB LED Matrix
- 015 - MicroPython TechNotes: Neopixel 16
- 014 - MicroPython TechNotes: 8x8 Dot Matrix Display (I2C)
- 013 - MicroPython TechNotes: 8x16 Dot Matrix Display (SPI)
- 012 - MicroPython TechNotes: 8x8 Dot Matrix Display (SPI)
- 011 - MicroPython TechNotes: 1.3 OLED Display
- 010 - MicroPython TechNotes: 0.96 OLED Display
- 009 - MicroPython TechNotes: 7 Segment Display
- 008 - MicroPython TechNotes: 16x2 LCD
- 007 - MicroPython TechNotes: RGB LED
- 006 - MicroPython TechNotes: Traffic Light LED Module
- 005 - MicroPython TechNotes: Gorilla Cell LED | MicroPython Hello World
- 004 - MicroPython TechNotes: Gorilla Cell I/O Devices
- 003 - MicroPython TechNotes: Gorillacell ESP32 Shield
- 002 - MicroPython TechNotes: Introduction for Gorillacell ESP32 Dev Kit
- 001 - MicroPython TechNotes: Get Started with MicroPython
- 000 - MicroPython TechNotes: Unboxing Gorillacell ESP32 Development Kit
No comments yet!