024 - MicroPython TechNotes: Slider Switch
Introduction
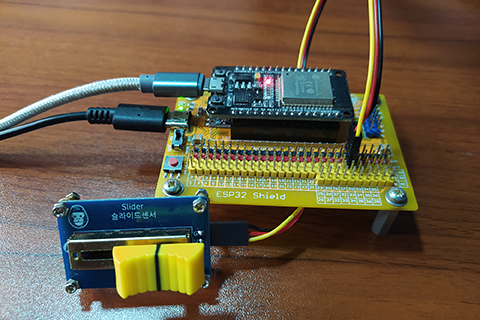
In this article, we will learn how to use Slider Switch with ESP32 using MicroPython programming language. A slider switch is basically a variable resistor that changes resistance according to slider wiper position. With that knowledge, we can used MicroPython’s ADC function to interpret slider switch position.
Pinout
- G – for the ground pin.
- V – for the supply voltage.
- S – for the signal pin.
Bill Of Materials
- ESP32 development board to serve as the brain for the experiment.
- ESP32 shield from Gorillacell ESP32 development kit to extend ESP32 pin to pin headers for easy circuit connection.
- 3-pin female-female dupont wires to connect slider switch to ESP32 shield pin headers.
- Slider Switch module from Gorillacell ESP32 development kit.
Hardware Instruction
- First, attach the ESP32 on top of the ESP32 shield by making sure that the pins are properly aligned and both USB ports are on the same side.
- Next, attach the dupont wires to the Slider module by following the color coding which is black for the ground, red for the VCC, and yellow for the signal pin.
- Next, attach the other end of the dupont wires to the ESP32 shield by matching the colors of the wires to the colors of the pin headers such as black to black, red to red, and yellow to yellow. For this experiment, I choose GPIO 32 to serve as the input signal pin from the Slider module.
- Next, power the ESP32 shield with external power supply with a type-C USB connector and make sure that the power switch is set to ON state.
- Lastly, connect the ESP32 to the computer through the USB cable with micro-USB type cable. Demo circuit is now ready.
Software Instruction
For this experiment, I prepared to example source code for you to try.
Copy and paste it to Thonny IDE.
Please feel free to modify it and adapt according to your needs.
Happy tinkering.
Video Demonstration
Call To Action
For any concern, write your message in the comment section.
You might also like to support my journey on Youtube by Subscribing. Click this to Subscribe to TechToTinker.
Thank you and have a good days ahead.
See you,
– George Bantique | tech.to.tinker@gmail.com
Source Code
1. Example # 1, basics of reading an analog input such as the slider switch:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC
5from time import sleep_ms
6
7p32 = Pin(32, Pin.IN)
8slider = ADC(p32)
9slider.atten(ADC.ATTN_11DB)
10
11while True:
12 print(slider.read())
13 sleep_ms(300)
2. Example # 2, simple application in using a slider switch:
1# More details can be found in TechToTinker.blogspot.com
2# George Bantique | tech.to.tinker@gmail.com
3
4from machine import Pin, ADC, PWM
5from time import sleep_ms
6
7p32 = Pin(32, Pin.IN)
8slider = ADC(p32)
9slider.atten(ADC.ATTN_11DB)
10
11boot = Pin(0, Pin.IN)
12led = Pin(2, Pin.OUT)
13
14red = PWM(Pin(25, Pin.OUT))
15grn = PWM(Pin(26, Pin.OUT))
16blu = PWM(Pin(27, Pin.OUT))
17red.freq(60)
18grn.freq(60)
19blu.freq(60)
20
21r = 0
22g = 0
23b = 0
24steps = 0
25
26def map(x, in_min, in_max, out_min, out_max):
27 # This will not handle x value greater than in_max or
28 # x value less than in_min
29 return int((x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min)
30
31while True:
32 if steps==0:
33 # Set the RGB from r, g, b values
34 red.duty(r)
35 grn.duty(g)
36 blu.duty(b)
37 if boot.value()==0:
38 steps = 1 # go to setting red
39 print('RGB are {} {} {}'.format(r, g, b))
40
41 elif steps==1:
42 # Set the red color
43 r = map(slider.read(), 0, 4095, 0, 255)
44 red.duty(r)
45 grn.duty(0)
46 blu.duty(0)
47 if boot.value()==0:
48 steps = 2 # go to setting green
49 print('R is set to {}'.format(r))
50
51 elif steps==2:
52 # Set the green color
53 g = map(slider.read(), 0, 4095, 0, 255)
54 red.duty(0)
55 grn.duty(g)
56 blu.duty(0)
57 if boot.value()==0:
58 steps = 3 # go to setting blue
59 print('G is set to {}'.format(g))
60
61 elif steps==3:
62 # Set the blue color
63 b = map(slider.read(), 0, 4095, 0, 255)
64 red.duty(0)
65 grn.duty(0)
66 blu.duty(b)
67 if boot.value()==0:
68 steps = 0 # write the r,g,b to RGB LED
69 print('B is set to {}'.format(b))
70
71 sleep_ms(300)
72 if steps==1 or steps==2 or steps==3:
73 led.value(not led.value())
74 else:
75 led.value(0)
References And Credits
- Purchase your Gorillacell ESP32 development kit at: http://gorillacell.kr
Posts in this series
- 049 - MicroPython TechNotes: MP3 Player
- 048 - MicroPython TechNotes: Analog Touch Sensor
- 047 - MicroPython TechNotes: E108 GPS
- 046 - MicroPython TechNotes: RF433 Transceivers
- 045 - MicroPython TechNotes: Infrared Transmitter
- 044 - MicroPython TechNotes: Infrared Receiver
- 043 - MicroPython TechNotes: ESP12E WiFi | External WiFi module
- 042 - MicroPython TechNotes: JDY-32 | Bluetooth Low Energy BLE
- 041 - MicroPython TechNotes: Bluetooth HC-06
- 040 - MicroPython TechNotes: Relay
- 039 - MicroPython TechNotes: Electromagnet
- 038 - MicroPython TechNotes: Buzzer
- 037 - MicroPython TechNotes: Servo Motor
- 036 - MicroPython TechNotes: Stepper Motor
- 035 - MicroPython TechNotes: Dual Motor Driver
- 034 - MicroPython TechNotes: DC Motors | Gear Motor and Fan Motor
- 033 - MicroPython TechNotes: TCS34725 RGB Color Sensor
- 032 - MicroPython TechNotes: BMP280 Sensor
- 031 - MicroPython TechNotes: TOF Distance Sensor
- 030 - MicroPython TechNotes: DS3231 RTC
- 029 - MicroPython TechNotes: HC-SR04 Ultrasonic Sensor
- 028 - MicroPython TechNotes: DHT11 Temperature and Humidity Sensor
- 027 - MicroPython TechNotes: Rotary Encoder
- 026 - MicroPython TechNotes: Light Dependent Resistor (LDR)
- 025 - MicroPython TechNotes: Joystick
- 023 - MicroPython TechNotes: Continuous Rotation Potentiometer
- 022 - MicroPython TechNotes: Potentiometer | Reading an Analog Input
- 021 - MicroPython TechNotes: Color Touch Sensor
- 020 - MicroPython TechNotes: Touch Sensor
- 019 - MicroPython TechNotes: Switch Module
- 018 - MicroPython TechNotes: Button | Reading an Input
- 017 - MicroPython TechNotes: LASER Module
- 016 - MicroPython TechNotes: RGB LED Matrix
- 015 - MicroPython TechNotes: Neopixel 16
- 014 - MicroPython TechNotes: 8x8 Dot Matrix Display (I2C)
- 013 - MicroPython TechNotes: 8x16 Dot Matrix Display (SPI)
- 012 - MicroPython TechNotes: 8x8 Dot Matrix Display (SPI)
- 011 - MicroPython TechNotes: 1.3 OLED Display
- 010 - MicroPython TechNotes: 0.96 OLED Display
- 009 - MicroPython TechNotes: 7 Segment Display
- 008 - MicroPython TechNotes: 16x2 LCD
- 007 - MicroPython TechNotes: RGB LED
- 006 - MicroPython TechNotes: Traffic Light LED Module
- 005 - MicroPython TechNotes: Gorilla Cell LED | MicroPython Hello World
- 004 - MicroPython TechNotes: Gorilla Cell I/O Devices
- 003 - MicroPython TechNotes: Gorillacell ESP32 Shield
- 002 - MicroPython TechNotes: Introduction for Gorillacell ESP32 Dev Kit
- 001 - MicroPython TechNotes: Get Started with MicroPython
- 000 - MicroPython TechNotes: Unboxing Gorillacell ESP32 Development Kit
No comments yet!