Interfacing Infrared to Arduino Uno
Introduction
I am thinking if it is possible to create a single remote controller for the appliances in our home. So I do some search in google on how to interface infrared to a microcontroller. To my surprised it was very easy. But for the meantime while I do not have the materials, here is the infrared decoder. This could decode infrared code sent by our remote controllers, and ofcourse every remote controller has a different set of code. With the power of microcontroller, it is possible to change the set of IR code by just pressing a single button switch. LEDs will be use as indicators. Hello peeps. I am George, welcome to tech-to-tinker channel, where technology is explored and shared.
And today, I am going to show you my tinkering with the Infrared reciever to Arduino Uno
Bill Of Materials
- Arduino Uno
- Keypad/LCD shield
- Infrared receiver
- Some jumper wires
Hardware Instruction
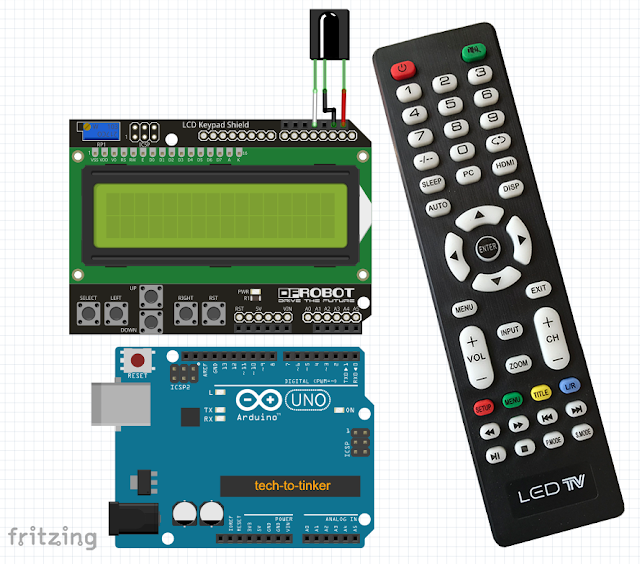
- Connect the Keypad/LCD shield at the top of Arduino Uno board. Only the LCD of the shield will be use for this tinkering.
- Connect the Infrared receiver as follows from Right-to-Left:
- VCC connected to Arduino 5V (red wire)
- GND connected to Arduino GND (black wire)
- Output pin connected to digital pin 3 of Arduino (white wire)
- Upload the sketch provided.
- Enjoy.
Video Demonstration
Source Code
1
2#include "IRremote.h"
3#include "LiquidCrystal.h"
4
5LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
6
7const int RECV_PIN = 3;
8IRrecv irrecv(RECV_PIN);
9decode_results results;
10unsigned long key_value = 0;
11
12void setup(){
13 Serial.begin(9600);
14 irrecv.enableIRIn();
15 irrecv.blink13(true);
16
17 // LCD
18 lcd.begin(16, 2);
19 lcd.setCursor(0, 0);
20 lcd.print(" tech-to-tinker ");
21 lcd.setCursor(0, 1);
22 lcd.print(" IR Code:");
23}
24
25void loop(){
26 if (irrecv.decode(&results)){
27 if (results.value == 0XFFFFFFFF)
28 results.value = key_value;
29
30 switch(results.value){
31 /*
32 case 0xFDD22D:
33 Serial.println("CH-");
34 lcd.setCursor(10, 1); // set the position
35 lcd.print(" ");
36 lcd.setCursor(10,1);
37 lcd.print("CH-");
38 break;
39 case 0xFD52AD:
40 Serial.println("CH+");
41 lcd.setCursor(10, 1); // set the position
42 lcd.print(" ");
43 lcd.setCursor(10,1);
44 lcd.print("CH+");
45 break;
46 case 0xFD6A95:
47 Serial.println("<<");
48 lcd.setCursor(10, 1); // set the position
49 lcd.print(" ");
50 lcd.setCursor(10,1);
51 lcd.print("<<");
52 break;
53 case 0xFDEA15:
54 Serial.println(">>");
55 lcd.setCursor(10, 1); // set the position
56 lcd.print(" ");
57 lcd.setCursor(10,1);
58 lcd.print(">>");
59 break ;
60 case 0xFD8A75:
61 Serial.println(">||");
62 lcd.setCursor(10, 1); // set the position
63 lcd.print(" ");
64 lcd.setCursor(10,1);
65 lcd.print(">||");
66 break ;
67 case 0xFD926D:
68 Serial.println("Vol-");
69 lcd.setCursor(10, 1); // set the position
70 lcd.print(" ");
71 lcd.setCursor(10,1);
72 lcd.print("Vol-");
73 break ;
74 case 0xFD12ED:
75 Serial.println("Vol+");
76 lcd.setCursor(10, 1); // set the position
77 lcd.print(" ");
78 lcd.setCursor(10,1);
79 lcd.print("Vol+");
80 break ;
81 case 0XFDC837:
82 Serial.println("UP");
83 lcd.setCursor(10, 1); // set the position
84 lcd.print(" ");
85 lcd.setCursor(10,1);
86 lcd.print("UP");
87 break ;
88 case 0xFD8877:
89 Serial.println("LEFT");
90 lcd.setCursor(10, 1); // set the position
91 lcd.print(" ");
92 lcd.setCursor(10,1);
93 lcd.print("LEFT");
94 break ;
95 case 0xFD28D7:
96 Serial.println("DOWN");
97 lcd.setCursor(10, 1); // set the position
98 lcd.print(" ");
99 lcd.setCursor(10,1);
100 lcd.print("DOWN");
101 break ;
102 case 0XFD48B7:
103 Serial.println("RIGHT");
104 lcd.setCursor(10, 1); // set the position
105 lcd.print(" ");
106 lcd.setCursor(10,1);
107 lcd.print("RIGHT");
108 break ;
109 case 0xFD08F7:
110 Serial.println("ENTER");
111 lcd.setCursor(10, 1); // set the position
112 lcd.print(" ");
113 lcd.setCursor(10,1);
114 lcd.print("ENTER");
115 break ;
116 case 0xFDAA55:
117 Serial.println(">>|");
118 lcd.setCursor(10, 1); // set the position
119 lcd.print(" ");
120 lcd.setCursor(10,1);
121 lcd.print(">>|");
122 break ;
123 case 0xFD2AD5:
124 Serial.println("|<<");
125 lcd.setCursor(10, 1); // set the position
126 lcd.print(" ");
127 lcd.setCursor(10,1);
128 lcd.print("|<<");
129 break ;
130 case 0xFD4AB5:
131 Serial.println("STOP");
132 lcd.setCursor(10, 1); // set the position
133 lcd.print(" ");
134 lcd.setCursor(10,1);
135 lcd.print("STOP");
136 break ;
137 */
138 default:
139 Serial.println(results.value,HEX);
140 lcd.setCursor(10, 1); // set the position
141 lcd.print(" ");
142 lcd.setCursor(10,1);
143 lcd.print(results.value,HEX);
144 break ;
145 }
146 key_value = results.value;
147 irrecv.resume();
148 //delay(2000);
149 }
150}
Call To Action
If you find this blog post useful, please kindly support my blogs:
techtotinker.com
TechToTinker Youtube Channel
Posts in this series
- How to Get Started with ATTiny85 in Arduino IDE
- Tutorial: How to use MFRC522 RFID module using Arduino
- SOS Flasher Using Millis Function with Enable Switch
- Tutorial: How to use DS3231 RTC in Arduino
- Tutorial: How to use 0.96 OLED - a small and cute display
- Tutorial: Getting Started with the NRF24L01 | How to use | Arduino
- Tutorial: How to use SIM800L GSM Module for Controlling Anything | Arduino
- Tutorial: How to use Keypad | Text Entry Mode | Arduino
- Tutorial: How to use 4x4 Keypad | Arduino
- Project Idea: Arduino Voltmeter
- Project Idea: Door Lock Security | Arduino
- Multitasking with Arduino | Relay Timer Controller | using millis
- Tutorial Understanding Blink Without Delay | How to millis
- Arduino Simple LCD Menu
- How to use SIM800L GSM Module using Arduino | Make or Answer Voice Calls
- Tutorial: How to Use Arduino Uno as HID | Part 2: Arduino Mouse Emulation
- Tutorial: How to Use Arduino Uno as HID | Part 1: Arduino Keyboard Emulation
- Tutorial: How to use SIM800L DTMF to Control Anything | Arduino
- Tutorial: Arduino EEPROM
- How to use SIM800L GSM Module | Arduino | Send and Receive SMS
- 16x2 LCD Menu for Arduino
- Tutorial: Arduino GPIO | How to use Arduino Pins
- MIT App Inventor for Arduino
- RC Car using L298N, HC-06, and Arduino Uno
- How to Use LCD Keypad Shield for Arduino
- How to Use Arduino Interrupts
- Project: Automatic Alcohol Dispenser
- TUTORIAL: How to use HC-SR04 Ultrasonic Sensor with Arduino
- Source Code: Astronomia Meme and Funeral Dance | melodies the Arduino way
- How to Get Started with L293D Motor Driver Shield with Arduino
- How to Get Started with L298N Motor Driver module using Arduino
- Part 2: Wav Music Player with Lyrics Using Arduino and SD Card
- Part 1: Wav Music Player Using Arduino Uno and SD Card
- How to Interface Stepper Motor to Arduino Uno
- How To Play MP3 Files on Arduino from SD Card
- What is Arduino Software Serial
- How to Interface SD card to Arduino (without SD card shield)?
- Playing Melodies Using Arduino
- 8 Degrees Of Freedom (DOF) Robot Using Arduino Uno
- How to Interface PS2 Controller to Arduino Uno
- Part 3: DF Player Mini Tinkering with Arduino Nano and LCD
- How to Interface HC-06 to Arduino
- How to make a Remote Control RC car using Arduino and HC-06 bluetooth module
- Part 2: DF Player Mini Tinkering with Arduino Nano
- Part 1: DF Player Mini - a mini cheap mp3 player
No comments yet!